Final result:
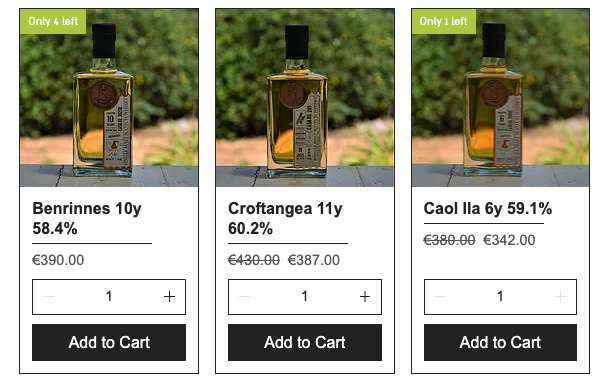
Hey fellow coders! In this post, I’ll show you how to create an auto-updating product ribbon that changes based on the product’s status in the store’s inventory. You will be able to set the limit of the quantity. Whenever a product’s stock reaches that specified minimum, a ribbon will be displayed on the product’s image with the current quantity. This specified minimum will be defined as the variable named MINIMUM_QUANTITY.
A few notes before starting:
This feature will be applied only to products that do not have variants. So a product that has 2 variants with quantities 1 and 0 will not show an ‘Only 1 left’ ribbon because it has another variant.
You won’t be able to store the old ribbon that was displayed before the item reached the MINIMUM_QUANTITY.
So let’s begin:
To enable this feature, add the following events.js and stockIndicatorRibbon.js files to your site’s backend, which will apply from the next inventory change of a non-variant product.
To control the MINIMUM_QUANTITY, change the value of the MINIMUM_QUANTITY variable in stockIndicatorRibbon.js. For example, if you want to display that a product has only 3 items left or less, set MINIMUM_QUANTITY to 3.
events.js:
The events used here are wixStores_onInventoryVariantUpdated and wixStores_onInventoryItemUpdated.
The onInventoryVariantUpdated() event fires whenever there is a change in a variant’s quantity. This event will give us the old quantity before the event fired, and the new quantity after the event fired.
The second event, onInventoryItemUpdated(), fires whenever there is a change to a product’s inventory settings—for example, if we stopped tracking the quantity of a certain product.
Create 2 backend files: events.js (this is a mandatory name, events won’t fire if we give this file another name - read more about it here) and stockIndicatorRibbon.js.
//event.js:
import wixData from 'wix-data';
import { updateRibbon, setDefaultRibbon } from 'backend/stockIndicatorRibbon'
const VARIANT_ID_OF_PRODUCT_WITH_NO_VARAINTS = '00000000-0000-0000-0000-000000000000'
export async function wixStores_onInventoryVariantUpdated(event) {
console.log(event);
const variant = event.variants[0];
if (variant.id === VARIANT_ID_OF_PRODUCT_WITH_NO_VARAINTS) {
const newItemQuantity = variant.newValue.quantity;
const oldItemQuantity = variant.oldValue.quantity;
return updateRibbon(event.productId, newItemQuantity, oldItemQuantity);
}
}
export async function wixStores_onInventoryItemUpdated(event) {
if (!event.trackInventory) {
const inventoryItem = await wixData.get('Stores/InventoryItems', event.inventoryItemId);
const variant = inventoryItem.variants[0];
if ( variant.variantId === VARIANT_ID_OF_PRODUCT_WITH_NO_VARAINTS){
return setDefaultRibbon(event.externalId);
}
}
}
stockIndicatorRibbon.js:
Since we’re handling only non-variant products, we handle variants with ID '00000000-0000-0000-0000-000000000000' which is the default ID for non-variant products.
We are collecting the old product quantity (before the event fired) and the new product quantity, then updating it in the function updateRibbon(), which you can see in stockIndicatorRibbon.js.
If inventory tracking is disabled, we want to clear the ribbon from non-relevant quantities. We perform this in the onInventoryItemUpdated event.
//stockIndicatorRibbon.js
import wixStoresBackend from 'wix-stores-backend';
import wixData from 'wix-data'
const MINIMUM_QUANTITY = 6;
export async function updateRibbon(productId, newItemQuantity, oldItemQuantity) {
if (isInMinimumQuantity(newItemQuantity)) {
return setNewRibbon(productId, newItemQuantity)
} else if (newItemQuantity === 0 ||
isInMinimumQuantity(oldItemQuantity) && !isInMinimumQuantity(newItemQuantity)) {
return setDefaultRibbon(productId);
}
}
function isInMinimumQuantity(quantity) {
return (quantity > 0 && quantity <= MINIMUM_QUANTITY);
}
async function setNewRibbon(productId, productVariantQuantity) {
return wixStoresBackend.updateProductFields(productId, { "ribbon": `Only ${productVariantQuantity} left` })
}
export async function setDefaultRibbon(productId) {
const productItem = await wixData.get('Stores/Products', productId);
if (productItem.ribbon !== '') {
return wixStoresBackend.updateProductFields(productId, { "ribbon": '' })
}
}
updateRibbon() is the main function here. It gets its info from the onInventoryVariantUpdated() event and updates the ribbon according to the current quantity.
If the product's new quantity is lower than the MINIMUM_QUANTITY limit, we should update the ribbon to the new quantity.
If the product’s new quantity is 0, we will clear the ribbon, because there are no longer available items.
If the product’s new quantity is above the MINIMUM_QUANTITY limit, and the old quantity was lower than the limit (when you restock the product), we will clear the ribbon as well.
You can change the text in the setNewRibbon() function from ‘Only X left!’ to whatever you want the ribbon text to display.
The default ribbon text is “ “, meaning no ribbon, and will be applied once the quantity is above the MINIMUM_QUANTITY. Of course you can set it to anything you like in the setDefaultRibbon() function.
Now you can publish your site and enjoy this cool feature! Good luck :)