Hello, Wanted to see if anyone has experience with Triggered Emails and has some suggestions or what I am missing. Basically, I am stuck on triggering an email for a custom price quote form that I created. I have checked all the documentation and a large amount of forum posts. I have tried for hours to rework my code but no luck. Would someone be able to point me in the right direction on what I have done wrong or am missing? Here is a stripped-down version of my code to save on post length (with less inputs etc.)
import wixData from 'wix-data';
import { contacts } from 'wix-crm';
import { triggeredEmails } from 'wix-crm';
//Does the quote calculation using number input fields
$w.onReady(function () {
$w("#numberRegular").onChange(Price);
$w("#numberSmall").onChange(Price);
});
//Declares price for each input
function Price() {
let regularPrice = 100
let smallPrice = 71
//Declares input name so they can be calculated easier
let Regular = $w('#numberRegular').value
let Small = $w('#numberSmall').value
//Calculates how many chosen by price defined above and displays it in an another input field. Works great!
$w("#priceTotal").value = (Regular * regularPrice) + (Small * smallPrice);
}
//Inserts form values into database after Submit button is clicked works great.
export function submit_click(event) {
let quote = {
"regular": $w("#numberRegular").value,
"small": $w("#numberSmall").value,
"name": $w("#name").value,
"lastName": $w("#name").value,
"email": $w("#email").value,
"phoneNumber": $w("#phone").value,
"address": $w("#address").value,
"price": $w("#priceTotal").value,
};
wixData.insert("QuoteCollection", quote)
//Clears form fields immediately after
$w("#numberRegular").value = '';
$w('#name').value = '';
$w('#lastName').value = '';
$w("#numberSmall").value ='';
$w("#description").value ='';
$w("#email").value ='';
$w("#phone").value ='';
$w("#address").value ='';
$w("#priceTotal").value ='';
//Resets form fields so they aren't in an error state from being cleared
$w("#numberRegular").resetValidityIndication();
$w('#name').resetValidityIndication();
$w('#lastName').resetValidityIndication();
$w("#numberSmall").resetValidityIndication();
$w("#description").resetValidityIndication();
$w("#email").resetValidityIndication();
$w("#phone").resetValidityIndication();
$w("#address").resetValidityIndication();
$w("#priceTotal").resetValidityIndication();
//Supposed to create a new contact then send triggered email - This is where I am having trouble
const contactInfo = {
name: { first: $w("#name").value , last: $w("#lastName").value},
emails: [ { email: $w("#email").value } ]
};
contacts.appendOrCreateContact(contactInfo)
.then((result) => {
let contactId = result.contactId
console.log(contactId, "this is the contact ID")
triggeredEmails.emailContact('TriggeredEmailQuote', contactId, {
//These are the variables in the triggered email I have setup already
variables: {
TotalPrice: $w("#priceTotal").value,
Description: $w("#description").value,
Regular: $w("#numberRegular").value,
Small: $w("#numberSmall").value
}
})
.then(() => {
})
.catch((err) => {
console.log(err)
});
});
}
Here are the errors I am getting from my console Logs.
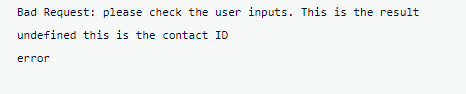
The quote calculator works great and shows the price based on the numbered inputs. It adds everything to the collection after the submit button, however it does not create a new contact and does not send the triggered email. Any help would be incredibly appreciated. I was thinking I am missing an afterInsert or something but not sure what. Thanks in advance!
I would try to run the create contact in the backend and to print the contact info before the create to make sure it is correct.
Why do you empty the fields before creating the contact? try to do it after if you need to